Bootstrap Tutorial | Learn Responsive Web Design & Front-End Development
Explore our Bootstrap Tutorial, the most popular front-end framework for web development. Learn how to build responsive, mobile-first websites using HTML, CSS, and JavaScript. Discover Bootstrap’s grid system, UI components, navigation bars, modals, cross-browser compatibility, and accessibility features. Ideal for beginners and professionals, this guide helps you create sleek and modern web designs efficiently.
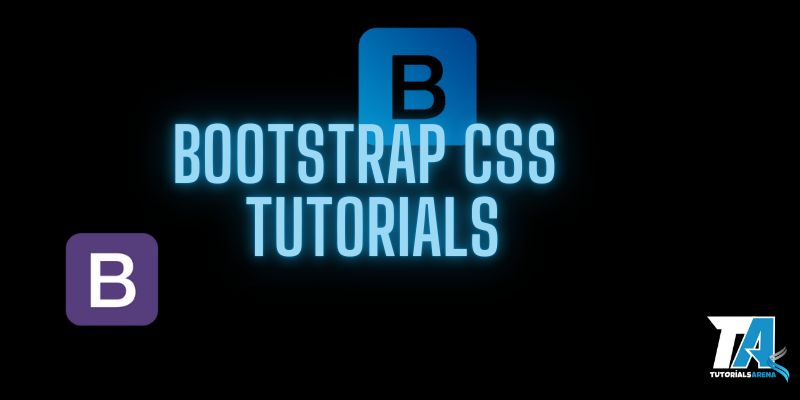
Bootstrap Tutorial
Bootstrap is the most popular front-end framework for web development. It is a sleek, intuitive, and powerful mobile-first framework that simplifies web design using HTML, CSS, and JavaScript.
Why Learn Bootstrap?
- Responsive Design: Bootstrap is built with a mobile-first approach, ensuring that web applications work well across different screen sizes.
- Time-Saving: It provides predefined CSS and JavaScript components such as grids, buttons, navigation bars, and modals, reducing development time.
- Consistent Appearance: Bootstrap offers a set of predefined styles and themes for a professional and cohesive design.
- Cross-Browser Compatibility: It ensures a seamless experience across different web browsers.
- Community and Support: A large community provides extensive documentation, forums, and online resources.
- Accessibility: Follows web development standards, making websites accessible to users with disabilities.
- Continuous Updates: Regular updates bring new features, bug fixes, and performance enhancements.
First Program Using Bootstrap
Below is a simple Bootstrap example to get you started. Copy and paste this code into an HTML file and run it in your browser.
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Bootstrap Example</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h1 class="text-center text-primary mt-5">Welcome to Bootstrap</h1>
<p class="text-center">This is a simple Bootstrap example.</p>
<button class="btn btn-primary d-block mx-auto">Click Me</button>
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Topics Covered in This Tutorial
- Bootstrap Basic Structure
- Bootstrap Layout
- Bootstrap Components
- Bootstrap Forms
- Bootstrap Grids
- Bootstrap Utilities
- Bootstrap Examples
Prerequisites
Before starting this tutorial, you should have a basic understanding of HTML and CSS. If not, it is recommended to go through introductory tutorials on these topics.
Who Can Learn Bootstrap?
This tutorial is designed for anyone interested in web development. Whether you are a beginner or an experienced developer, Bootstrap makes front-end development easier and more efficient.
Need Help?
If you encounter any issues while learning Bootstrap, feel free to explore community forums, documentation, and online resources for further guidance.
Core Bootstrap Concepts
- Bootstrap Tutorial
- What is Bootstrap?
- Bootstrap Example
- Bootstrap CDN
- Bootstrap Spinners
- Bootstrap Star Rating
- Bootstrap Tooltip
- Bootstrap Alternatives
- Bootstrap Container
- Bootstrap Jumbotron
- Bootstrap Button
- Bootstrap Grid
- Bootstrap Table
- Bootstrap Form
- Bootstrap Alert
- Bootstrap Wells
- Bootstrap Badges and Labels
- Bootstrap Panels
- Bootstrap Pagination
- Bootstrap Pager
- Bootstrap Image
- Bootstrap Glyphicon
- Bootstrap Carousel
- Bootstrap Progress Bar
- Bootstrap List Group
- Bootstrap Dropdown
- Bootstrap Collapse
- Bootstrap Tabs and Pills
- Bootstrap Navbar
- Bootstrap Inputs
- Bootstrap Modals
- Bootstrap Popover
- Bootstrap Scrollspy
- Bootstrap Border
- Bootstrap Clearfix
- Bootstrap Close Icons
- Bootstrap Colors
- Bootstrap Flexbox
- Bootstrap Display Property
- Bootstrap Image Replacement
- Bootstrap Invisible Content
- Bootstrap Position
- Bootstrap Responsive Helpers
- Bootstrap Screen Readers
- Bootstrap Sizing
- Bootstrap Spacing
- Bootstrap Typography
- Bootstrap Picker
- Add to Cart Button
- Change Bootstrap Datepicker Format
- Bootstrap Datepicker in Angular
- Setting Datepicker in Bootstrap
- Bootstrap Datepicker onChange Event
- Bootstrap Datepicker in Laravel
- Bootstrap Timepicker with DateTimePicker
- Load More Feature
- Bootstrap Typeahead Autocomplete
- Bootstrap 3 Footer
- Bootstrap Collapse in Angular
- Bootstrap Rating Widget
- Bootstrap Multiselect
- Bootstrap Growl Notifications
- Bootstrap 3 Sticky Header
- Responsive Images in Bootstrap
- Bootstrap Protocol
- Aligning Buttons in Bootstrap
- Changing Navbar Color
- Creating Charts with Bootstrap
- Horizontal Scrolling in Bootstrap
- Bootstrap MCQ Quiz
- HTML Interview Questions
- CSS Interview Questions
- jQuery Interview Questions
- JavaScript Interview Questions
- AJAX Interview Questions