Java Tutorial: Learn Java Programming from Beginner to Advanced
Become a Java expert with our comprehensive tutorial. Learn Java programming concepts from scratch, with clear explanations, practical examples, and exercises. This tutorial is perfect for beginners and experienced programmers alike.
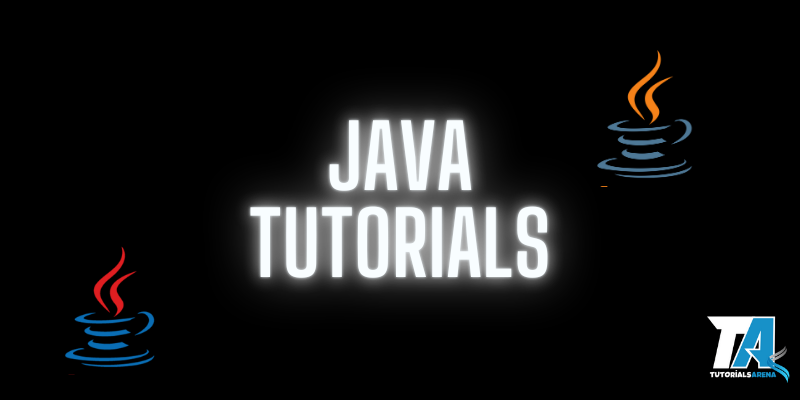
Java Tutorial
This Java tutorial has been written for beginners to advanced programmers who are striving to learn Java programming. Numerous practical examples have been provided to explain the concepts in simple and easy steps. This tutorial has been prepared and reviewed by experienced Java programmers at Tutorials Point, ensuring its usefulness for both students and Java developers.
After completing this tutorial, you will find yourself at a moderate level of expertise in Java programming, from where you can elevate yourself to the next levels.
What is Java?
Java is a popular high-level, object-oriented programming language that was originally developed by Sun Microsystems and released in 1995. Currently, Java is owned by Oracle, and more than 3 billion devices run Java. It runs on various platforms like Windows, Mac OS, and the various versions of UNIX. Java is used to develop numerous types of software applications, including desktop apps, mobile apps, web apps, games, and more.
Java follows the principle of "Write Once, Run Anywhere" (WORA), meaning that compiled Java code can run on any platform that supports Java without the need for recompilation.
Java First Example
The first example in Java is to print "Hello, World!" on the screen. Here's a simple Java code to achieve that:
Syntax
public class MyFirstJavaProgram {
/* This is my first Java program.
* This will print 'Hello, World!' as the output
*/
public static void main(String []args) {
System.out.println("Hello, World!"); // prints Hello, World!
}
}
Output
Hello, World!
Java Features
- Object-Oriented: Everything in Java is an object. It supports OOP principles like inheritance, encapsulation, and polymorphism.
- Platform Independent: Java is compiled into platform-neutral byte code, which is executed by the JVM on different platforms.
- Easy to Learn: Java inherits features from C and C++, making it easier for developers familiar with those languages to learn Java.
- Secure: Java provides automatic memory management and garbage collection, making it safer and more secure.
- Multithreading: Java has inbuilt support for multithreading, making it efficient for tasks like concurrent processing.
- High Performance: Java uses the Just-In-Time (JIT) compiler, which enhances performance by compiling code at runtime.
- Portable: Java code can run on various platforms without modification, making it highly portable.
Java Applications
Java is used in many areas, such as:
- Enterprise solutions
- Game development
- Secure web development
- Embedded systems
- Mobile application development
- Big data applications
Java Platforms (Editions)
There are four editions of Java:
- Java SE (Standard Edition): Used for developing desktop and server applications.
- Java EE (Enterprise Edition): Used for building web applications.
- Java ME (Micro Edition): Used for mobile and embedded applications.
- JavaFX: Used for creating lightweight, rich user interfaces.
Java Jobs & Opportunities
Java developers are in high demand, with salaries ranging up to $120,000 annually for professionals with 3-5 years of experience. Companies like Google, Microsoft, Facebook, IBM, Amazon, and many others actively seek Java programmers.
Why Learn Java?
Java is essential for aspiring software developers due to its simplicity, versatility, high demand, and excellent job prospects. Learning Java will open doors to numerous career opportunities, including desktop, mobile, and web application development.
Prerequisites to Learn Java
Before diving into Java, it's assumed that readers have a basic understanding of programming concepts like variables, syntax, and commands. Having experience with any programming environment will also be beneficial.
Learn Java by Examples
This tutorial provides a set of practical Java examples to help you master various concepts.
Getting Started with Java
Start your Java learning journey by understanding the basics, environment setup, and then proceed with the topics provided in this tutorial.
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java - Java vs C++
- Java - JVM
- Java - JDK, JRE, JVM
- Java - Hello World
- Java - Environment Setup
- Java - Basic Syntax
- Java - Naming Convention
- Java - Variable Types
- Java - Variable Identifiers
- Java - Declare Multiple Variables
- Java - Variable Scope
- Java - Printing Variables
- Java - Variable Examples
- Java - Basic Data Types
- Java - Number Data Types
- Java - Boolean Data Types
- Java - Character Data Types
- Java - Non Primitive Data Types
- Java - Data Types Examples
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
- Java - Date Time
- Java - Linked Lists
- Java - Linked Lists
- Java - Loop Control
- Java - Decision Making
- Java - If-Else Statement
- Java - If-Else Statement
- Java - Switch Statement
- Java - For Loop
- Java - Foreach Loop
- Java - Nested For Loop
- Java - While Loop
- Java - Do While Loop
- Java - Break Statement
- Java - Continue Statement
- Java - Conditions Examples
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Object Cloning
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Method Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Lang Enum
- Java - Enum Constructor
- Java - Enum String
- Java - Lang Number
- Java - Lang Boolean
- Java - Characters
- Java - Arrays
- Java - Looping in Arrays
- Java - Multidimension Arrays
- Java Arrays Class
- Java - Lang Math
- Java - File Class
- Java - Create File
- Java - Write File
- Java - Read File
- Java - Delete File
- Java - Directories
- Java - Files IO
- Java - Exceptions
- Java - Transient Variable
- Java - Try Catch Block
- Java - Try With Resources
- Java - Multi Catch Block
- Java - Nested Try Block
- Java - Finally Block
- Java - Throw Exception
- Java - Exception Propagation
- Java - Builtin Exceptions
- Java - Custom Exception
- Java - Multithreading
- Java - Thread Multitasking
- Java - Thread Life Cycle
- Java - Create Thread
- Java - Start Thread
- Java - Join Thread
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pool
- Java - Main Thread
- Java - Thread Priority
- Java - Thread SLeep
- Java - Daemon Thread
- Java - Lang ThreadGroup
- Java - Shutdown Hook
- Java - Thread Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Thread Communication
- Java - Thread Deadlock
- Java - Interrupting Thread
- Java - Thread Control
- Java - Thread Runtime Class
- Java - Reentrant Monitor
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL
- Java - URLConnection
- Java Network Datagram
- Java - InetAddress Class
- Java - HttpURLConnection
- Java - Socket
- Java - Generics
- Java - Collections
- Java - Collection Interface
- Java - List Interface
- Java - HashMap
- Java - HashSet
- Java - Util Queue
- Java - Map Interface
- Java - SortedMap Interface
- Java - Set Interface
- Java - SortedSet Interface
- Java - Data Structures
- Java - Enumeration Interface
- Java - Using Iterator
- Java - Using Comparator
- Java - Using Comparable
- Java - Command Line Args
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Documentation
- Java - Autoboxing & Unboxing
- Java - File Mismatch Method
- Java - REPL
- Java - Multi-release Jar
- Java - Private Interface Methods
- Java - Inner Class & Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64
- Java - Switch Expression
- Java - Teeing Collectors
- Java - Micro Benchmark
- Java - Text Blocks
- Java - Dynamic CDS
- Java - Z Garbage Collectors
- Java - NullPointerException
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record
- Java - Hidden Classes
- Java - Instanceof Pattern Matching
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - Just In Time (JIT) Compiler
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Strings Concatenation
- Java - Strings Number
- Java - Strings Special Chanracters
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Streams
- Java - DateTime API