C Programming: A Comprehensive Introduction
Embark on your C programming journey with this comprehensive introduction. Discover the fundamentals of C, its history, and its widespread applications. Understand why C remains a popular choice for efficient and flexible software development.
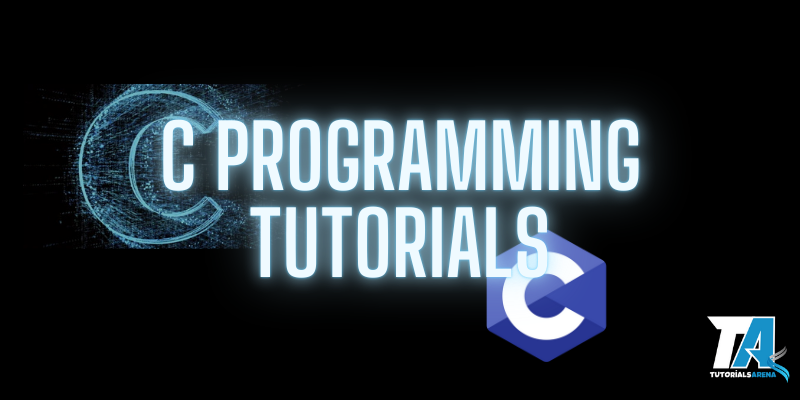
Introduction to C Programming
C is a general-purpose, procedural programming language created in 1972 by Dennis M. Ritchie at Bell Telephone Laboratories to develop the UNIX operating system. C remains one of the most widely used programming languages and is popular for its efficiency and flexibility. It frequently competes with Java in terms of popularity among modern software developers.
Why Learn C Programming?
Learning C programming is essential for students and professionals aiming to excel in software development. Here are some reasons to learn C:
- C is a structured programming language that helps build a strong foundation for mastering other languages.
- It allows you to write efficient code and create robust projects.
- C is a low-level language, providing direct interaction with hardware and memory, offering more control over system resources.
Explore our latest online courses and develop new skills at your own pace. Enroll today and become a certified expert to boost your career.
Key Facts about C Programming
C is widely recognized as the most popular system programming language. Here are a few interesting facts about C:
- C was invented to create the UNIX operating system, which was written entirely in C.
- C is the successor of the B programming language, developed in the early 1970s.
- The C language was formalized in 1988 by the American National Standards Institute (ANSI).
Hello World Program in C
Here’s a simple "Hello World" program to give you a taste of C programming. You can run it in your preferred compiler or editor.
Syntax
#include <stdio.h>
int main() {
/* My first program in C */
printf("Hello, World! \n");
return 0;
}
Applications of C Programming
C was originally designed for system development, particularly for writing operating systems. It was chosen because of its ability to produce code that runs as fast as assembly language. Some common applications of C include:
- Operating Systems
- Language Compilers
- Assemblers
- Text Editors
- Print Spoolers
- Network Drivers
- Modern Software Programs
- Databases
- Language Interpreters
- System Utilities
Audience
This tutorial is designed for software programmers who want to learn C programming from scratch. By the end of this guide, you will have a solid understanding of the C programming language, allowing you to advance your skills further.
Prerequisites
Before diving into this tutorial, it’s helpful to have a basic understanding of programming concepts and computer terminologies. Familiarity with any other programming language will also make it easier to grasp C programming concepts more quickly.
- C - Home
- C - Introduction
- C - Getting Started
- C - History
- C - Environment Setup
- C - Syntax
- C - Hello World
- C - Features
- C - Program Structure
- C - Statements
- C - Output
- C - Comments
- C - Variables
- C - Variable Formats
- C - Changing Variables
- C - Multiple Variables
- C - Variable Names
- C - Variables in Real Life
- C - Data Types
- C - Character Data Types
- C - Number Data Types
- C - Decimal Data Types
- C - Size of Data Types
- C - Data Types in Real Life
- C - Type Conversion
- C - Constants
- C - Escape Sequences
- C - Format Specifiers
- C - Literals
- C - Operators
- C - Arithmetic Operators
- C - Assignment Operators
- C - Bitwise Operators
- C - Increment and Decrement Operators
- C - Logical Operators
- C - Operator Precedence
- C - Relational Operators
- C - sizeof Operator
- C - Ternary Operator
- C - Unary Operators
- C - Booleans
- C - Booleans in Real Life
- C - Conditions
- C - If Statement
- C - Else Statement
- C - If-Else Statement
- C - Else If Statement
- C - Short Hand Condition
- C - Conditions in Real Life
- C - Switch Statement
- C - Nested Switch
- C - While Loop
- C - Do While Loop
- C - While Loop in Real Life
- C - For Loop
- C - Nested For Loop
- C - For Loop in Real Life
- C - Go To Statement
- C - Infinite Loop
- C - Break and Continue
- C - Arrays
- C - Array Size
- C - Arrays in Real Life
- C - Multidimensional Arrays
- C - Strings
- C - String Escape Sequences
- C - String Functions
- C - User Input
- C - Memory Address
- C - Pointers
- C - Pointers and Arrays
- C - Application of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Returning Pointer from Functions
- C - Passing Pointers to Functions
- C - Function Pointers
- C - Pointers to Arrays
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointers vs Arrays
- C - Char Pointers and Functions
- C - Null Pointer
- C - Void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far, and Huge Pointers
- C - Initializing Pointer Arrays
- C - Pointers and Multidimensional Arrays
- C - Functions
- C - Function Parameters
- C - Scope
- C - Function Declaration
- C - Recursion
- C - Math Functions
- C - Files
- C - Writing to Files
- C - Reading from Files
- C - Structs
- C - Enums
- C - Memory Management
- C - Memory Allocation
- C - Memory Access
- C - Memory Reallocation
- C - Memory Deallocation
- C - Memory in Real Life
- C - Reference Keywords
- C - Reference stdio.h
- C - Reference stdlib.h
- C - Reference string.h
- C - Reference math.h
- C - Reference ctype.h
- C - Examples
- C - Real Life Examples