Mastering C#: A Comprehensive Programming Tutorial for All Levels
Learn C# programming from beginner to advanced concepts with our comprehensive tutorial. This guide covers key features, syntax, and practical examples, equipping you to build a wide range of applications across various platforms and domains.
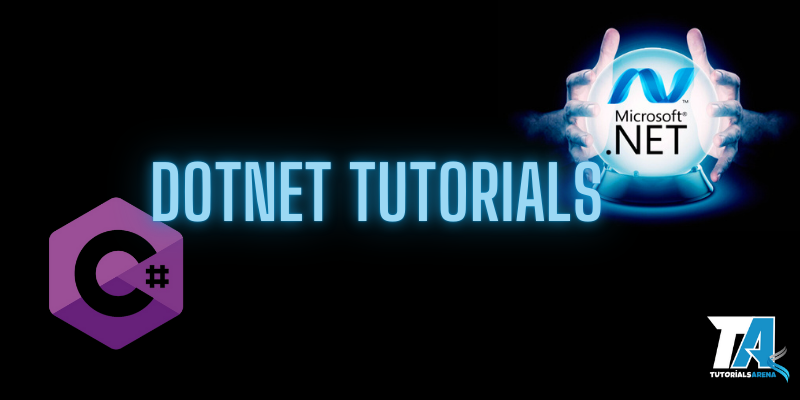
C# Tutorial
C# is a modern, general-purpose, object-oriented programming language developed by Microsoft as part of its .NET initiative, led by Anders Hejlsberg. This tutorial will cover both basic and advanced C# programming concepts to help you become proficient in developing various applications, including desktop, web, mobile, cloud, and gaming applications.
Why C# - Need of C#
C# is both object-oriented and component-oriented, offering features that enhance its robustness, making it ideal for building secure and scalable applications. Some key features of C# include:
- Syntax: Its syntax is similar to other C-style languages (C, C++, Java), making it easy for developers to pick up.
- Object-Oriented: C# supports encapsulation, inheritance, and polymorphism, core principles of object-oriented programming.
- Compiled into Intermediate Language (IL): C# code is compiled into IL, executed on the Common Language Runtime (CLR), ensuring type safety, automated memory management (garbage collection), and exception handling.
- Platform Independence: With .NET Core or Mono, C# programs can run on various platforms like Windows, Linux, and macOS.
- Language Integrated Query (LINQ): LINQ allows type-safe data retrieval from databases, XML, and collections directly in C#.
- Asynchronous Programming: C# supports async and await keywords for writing non-blocking code.
- Rich Standard Library: The .NET library provides APIs for file I/O, networking, cryptography, and more.
C# Applications - Uses of C#
C# is versatile and used in a variety of applications:
- Desktop Applications: C# is used with frameworks like Windows Presentation Foundation (WPF) or Windows Forms to build desktop applications.
- Web Applications: With ASP.NET and ASP.NET Core, C# is widely used to develop web applications like e-commerce websites, content management systems, and web APIs.
- Mobile Applications: Xamarin enables cross-platform mobile application development for iOS, Android, and Windows Phone using C#.
- Game Development: C# is heavily used in game development with the Unity game engine, supporting 2D and 3D games for desktop, mobile, and consoles.
- Enterprise Software: C# is used to create business applications like CRM systems, ERP software, and other enterprise solutions.
- Cloud Services: C# is used to build cloud-based applications and services, particularly with Microsoft Azure.
- Internet of Things (IoT): C# is used in IoT application development, especially with Windows IoT Core and .NET Core frameworks.
- Machine Learning and Data Analysis: C# supports machine learning through libraries like ML.NET and tools like Microsoft Azure Machine Learning.
- Tools and Utilities: C# is often used to create development tools, system utilities, debuggers, and performance monitoring tools.
- Financial Applications: C# is common in financial software development, including trading platforms, risk management, and algorithmic trading systems.
Audience
This tutorial is designed for anyone who wants to learn C#, from beginners to advanced learners. It is particularly useful for developing applications in areas like Desktop Applications, Web Applications, Mobile Applications, Game Development, Cloud Services, IoT, Machine Learning, Data Analysis, Tools and Utilities, and more.
Prerequisites
Before starting this tutorial, it is recommended that you have a basic understanding of computer programming terminologies. Familiarity with C, C++, or object-oriented programming (OOP) concepts, or experience with other OOP languages like Java, will be beneficial.
- C# Home
- .NET Framework
- .NET Common Language Runtime
- .NET Framework Class Library
- C# Tutorial
- C# Example
- C# History
- C# Features
- Java vs C#
- C# Variables
- C# Data Types
- C# Operators
- C# Keywords
- C# If-Else
- C# Switch
- C# For Loop
- C# While Loop
- C# Do-While Loop
- C# Break Statement
- C# Continue Statement
- C# Goto Statement
- C# Function
- C# Call by Value
- C# Call by Reference
- C# Out Parameter
- C# Arrays
- Passing Array to Function in C#
- C# Multidimensional Array
- C# Jagged Array
- C# Params
- C# Array Class
- C# Command-Line Arguments
- C# Comments
- C# Object and Class
- C# Constructor
- C# Destructor
- C# 'this' Keyword
- C# Static
- C# Static Class
- C# Static Constructor
- C# Structs
- C# Enum
- C# Properties
- C# Inheritance
- C# Aggregation
- C# Member Overloading
- C# Method Overriding
- C# Base Keyword
- C# Polymorphism
- C# Sealed
- C# Abstract
- C# Interface
- C# Namespaces
- C# Access Modifiers
- C# Encapsulation
- C# Strings
- String.Clone
- String.Compare
- String.CompareOrdinal
- String.CompareTo
- String.Concat
- String.Contains
- String.Copy
- String.CopyTo
- String.EndsWith
- String.Equals
- String.Format
- String.GetEnumerator
- String.GetHashCode
- String.GetType
- String.GetTypeCode
- String.IndexOf
- String.Insert
- String.Intern
- String.IsInterned
- String.IsNormalized
- String.Normalize
- String.IsNullOrEmpty
- String.IsNullOrWhiteSpace
- String.Join
- String.LastIndexOf
- String.LastIndexOfAny
- String.PadLeft
- String.PadRight
- String.Remove
- String.Replace
- String.Split
- String.StartsWith
- String.Substring
- String.ToCharArray
- String.ToLower
- String.ToLowerInvariant
- String.ToString
- String.ToUpper
- String.ToUpperInvariant
- String.Trim
- String.TrimEnd
- String.TrimStart
- C# Exception Handling
- C# Try-Catch
- C# Finally
- C# User-Defined Exceptions
- C# Checked and Unchecked
- C# SystemException
- C# FileStream
- C# StreamWriter
- C# StreamReader
- C# TextWriter
- C# TextReader
- C# BinaryWriter
- C# BinaryReader
- C# StringWriter
- C# StringReader
- C# FileInfo
- C# DirectoryInfo
- C# Serialization
- C# Deserialization
- C# System.IO
- C# Collections
- C# List
- C# HashSet
- C# SortedSet
- C# Stack
- C# Queue
- C# LinkedList
- C# Dictionary
- C# SortedDictionary
- C# SortedList
- C# Generics
- C# Delegates
- C# Reflection
- C# Anonymous Function
- C# Multithreading
- C# Thread Life Cycle
- C# Thread Class
- C# Main Thread
- C# Threading Example
- C# Thread Sleep
- C# Thread Abort
- C# Thread Join
- C# Thread Name
- C# Thread Priority
- C# Thread Synchronization
- DateTime in C#
- Type Casting in C#
- ListBox Control in C#
- C# ReadLine Method
- DateTime in C#
- Type Casting in C#
- ListBox Control in C#
- C# ReadLine Method
- Design Patterns in C#
- C# Operator Overloading
- 3-Tier Architecture in C#
- C# Custom Attribute
- C# Game Development
- C# Rename File
- C# Validate Email
- C# WebClient
- C# XML Parser
- Parse JSON in C#
- Priority Queue in C#
- RestClient in C#
- Shadowing in C#
- Task vs Thread in C#
- Unmanaged Code in C#
- C# Socket Programming
- C# Unit Test Framework
- Convert DataTable to List in C#
- Escape Sequence in C#
- AES Encryption in C#
- HTML Encode in C#
- HTML to PDF in C#
- NHibernate in C#
- Any() in C#
- Advanced C#
- What is DLL in C#?
- GridView in C#
- .NET Architecture in C#
- C# Books for Beginners
- ORM in C#
- SignalR in C#
- Singleton Design Pattern in C#
- Throw vs Throw Ex in C#
- Error Logging in C#
- Throw Keyword in C#
- MSDN in C#
- C# Marshal
- C# Regex Tester
- Clean Architecture in C#
- Type of Assembly in C#
- Unity Container in C#
- SignalR in C#
- MSMQ in C#
- RabbitMQ in C#
- Resx File in C#
- What is IEnumerable in C#?
- Difference Between Readonly and Constant in C#
- How to Call Stored Procedure in C#
- Boxing and Unboxing in C#
- Convert Double to Int in C#
- Difference Between Struct and Class in C#
- Private Constructor in C#
- ForEach Loop in C#
- Volatile Keyword in C#
- IEnumerable vs IQueryable in C#
- StringCollection Class in C#
- Liskov Substitution Principle in C#
- Stack.Pop Method in C#
- Type.FindMembers Method in C#
- Char.TryParse Method in C#
- Char.Equals Method in C#
- File.AppendAllLines Method in C#
- File.GetLastWriteTimeUtc Method in C#
- Random.NextDouble Method in C#
- Console.SetIn Method in C#
- Math.BigMul Method in C#
- Single.IsFinite Method in C#
- Byte.MaxValue Field in C#
- SByte Struct Fields in C#
- ValueTuple Struct in C#
- DateTimeOffset.FromUnixTimeMilliseconds Method in C#
- Decimal.ToInt32 Method in C#
- Index Constructor in C#
- Byte.MinValue Field in C#
- Char.IsHighSurrogate Method in C#
- Char.IsSeparator Method in C#
- Char.IsWhiteSpace Method in C#
- Char.IsPunctuation Method in C#
- Char.IsSurrogate Method in C#
- Char.ConvertFromUtf32 Method in C#
- CharEnumerator.GetHashCode Method in C#
- CharEnumerator.ToString Method in C#
- Char.ToLowerInvariant Method in C#
- IndexOfAny Method in C#
- List.TrimExcess Method in C#
- Object.MemberwiseClone in C#
- Queue.Synchronized in C#
- Difference Between Properties and Indexers in C#
- Difference Between SortedList and SortedDictionary in C#
- Difference Between Static Constructors and Non-Static Constructors in C#
- Difference Between Delegates and Interfaces in C#
- Difference Between JavaScript and C#
- Program to Demonstrate the IList Interface in C#
- Program to Demonstrate the Use of FullName Property in C#
- How to Create a 7-Tuple (Septuple) in C#
- Implement 'Is' Functionality Without Using 'Is' Keyword in C#
- Array.BinarySearch Examples in C#
- Console.TreatControlCAsInput Property in C#
- Convert.ToSByte (String, IFormatProvider) Method in C#
- Decimal.GetHashCode Method in C#
- File.GetAttributes Method in C#
- Single.GetTypeCode Method in C#
- Single.IsPositiveInfinity Method in C#
- Stack.Clear Method in C#
- Uri.IsBaseOf(Uri) Method in C#
- CharEnumerator.Reset Method in C#
- Decimal.FromOACurrency Method in C#
- Math.IEEERemainder Method in C#
- Object.ReferenceEquals Method in C#
- OrderedDictionary.Item Property in C#
- File.SetCreationTime Method in C#
- Uri.EscapeDataString(String) Method in C#
- Uri.IsHexEncoding Method in C#
- Array.ConstrainedCopy Method in C#
- Array.TrueForAll Method in C#
- Buffer.BlockCopy Method in C#
- Check if the BitArray Is Read-Only in C#
- Decimal.Compare Method in C#
- Decimal.Floor Method in C#
- Decimal.ToSByte Method in C#
- Char.IsLetterOrDigit Method in C#
- Char.ToUpperInvariant(Char) Method in C#
- Single.IsNaN Method in C#
- Single.IsNegativeInfinity Method in C#
- StringBuilder.EnsureCapacity Method in C#
- Type.GetEnumUnderlyingType Method in C#
- Type.GetTypeHandle Method in C#
- File.SetLastWriteTimeUtc Method in C#
- GetTypeFromCLSID Method in C#
- GetTypeFromProgID Method in C#
- Difference Between 'is' and 'as' Operators in C#
- Difference Between C# and ASP.NET
- Difference Between Method Overriding and Method Hiding in C#
- Difference Between Python and C#
- Difference Between Console.Read and Console.ReadLine in C#
- Difference Between Hashtable and Dictionary in C#
- Difference Between Ref and Out Keywords in C#
- Difference Between Static Class and Singleton Instance in C#
- Difference Between Var and Dynamic in C#
- Difference Between Initialization and Instantiation in C#
- Differences Between Sealed Class and Static Class in C#
- Difference Between String Literal and String Object in C#
- Difference Between Func Delegate and Action Delegate in C#
- Difference Between System-Level and Application-Level Exceptions in C#
- Difference Between Multi-Dimensional Array and Jagged Array in C#
- Difference Between Lambda Expression and Delegate in C#
- Value Types vs. Reference Types in C#
- Getting an Enumerator for the Entire ArrayList in C#
- Exception Handling for Invalid Typecasting in Unboxing in C#
- Program to Check if a Class Is Sealed in C#
- Demonstrate the Use of CanRead Property in C#
- Demonstrate FailFast Method of Environment Class in C#
- Different Ways to Take Input and Print Float Value in C#
- Finding Index of First Element in Sequence in C#
- Get/Set Element at Specified Index in List in C#
- How to Compare Two ValueTuples in C#
- Get First Element of ValueTuple in C#
- Check if BitArray is Read-Only in C#
- Multicast Delegates in C#
- Null Coalescing Operator in C#
- SByte Keyword in C#
- Type.GetDefaultMembers Method in C#
- Type.IsAssignableFrom Method in C#
- Uri.Fragment Property in C#
- Default Interface Methods in C#
- Dependency Injection in C#
- Yield Keywords in C#
- Random.Next Method in C#
- Camel Case in C#
- Different Ways to Sort an Array in Descending Order in C#
- IDumpable Interface in C#
- Different Ways to Access Namespace in C#
- Use of CreateSubdirectory Method in C#
- Thread.CurrentThread Property in C#
- Type.GetInterfaces Method in C#
- Type.GetMembers Method in C#
- Type.GetNestedType Method in C#
- Get Minimum Value in SortedSet in C#
- HashSet
.ExceptWith(IEnumerable ) Method in C# - Console.SetWindowPosition Method in C#
- C# Program Using Method as a Condition in LINQ
- C# Program to Reverse Words in a Given String
- How to Combine Two Arrays Without Duplicate Values in C#
- How to Compare Enum Values in C#
- How to Remove All Characters From StringBuilder in C#
- How to Use Multiple Catch Clauses in C#
- How to Get Synchronized Access to the ListDictionary in C#
- How to Sort Object Array by Specific Property in C#
- How to Write Retry Logic in C#
- Console.CursorVisible in C#
- Console.ForegroundColor Property in C#
- Boolean.GetTypeCode Method in C#
- Hashtable.ContainsKey(Object) Method in C#
- SortedList.IndexOfValue(Object) Method in C#
- JsonConvert.DeserializeObject in C#
- Math.Round Method in C#
- MethodInfo.Invoke in C#
- Task.FromResult Method in C#
- Thread Pool in C#
- TimeSpan.FromDays Method in C#
- TimeSpan.FromTicks Method in C#
- Void Keyword in C#
- Queue.Contains Method in C#
- Queue.Enqueue Method in C#
- Stack.CopyTo Method in C#
- Stack.Contains Method in C#
- Stack
.TrimExcess Method in C# - SortedDictionary.Clear Method in C#
- SortedDictionary.Keys Property in C#
- SortedList.Clone Method in C#
- SortedList.ContainsKey Method in C#
- Type.GetCustomAttributes Method in C#
- Type.GetField Method in C#
- Type.IsSubclassOf Function in C#
- Type.GetArrayRank Method in C#
- Type.GetTypeFromHandle Method in C#
- Type.FindInterfaces Method in C#
- Uri.DnsSafeHost Property in C#
- Uri.ReferenceEquals Method in C#
- Uri.IsWellFormedOriginalString Method in C#
- Real-Time Examples of Factory Design Patterns in C#
- Abstract Factory Design Pattern in C#
- Facade Design Pattern in C# with Examples
- Structural Design Patterns in C#
- Immutable in C#
- Dictionary Initializer
- Pattern Matching in C#
- Tuples in C#
- Deconstruction in C#
- Single.IsInfinity Method in C#
- Single.CompareTo Method in C#
- Decimal.GetBits Method in C#
- CancellationToken in C#
- Encrypt and Decrypt Using Rijndael Key in C#
- Enumerable.Where Method in C#
- Interesting Facts About C#
- Task Parallel Library (TPL) in C#
- HybridDictionary Class in C#
- Optimization Tips for C# Code
- Inherit Documentation in C#
- Predicate Delegate in C#
- C# Programs
- C# Program for Producing a Filtered Sequence of Elements
- Fibonacci Series in C#
- Prime Number Program in C#
- Palindrome Program in C#
- Factorial Program in C#
- Armstrong Number in C#
- Sum of Digits Program in C#
- C# Program to Reverse a Number
- C# Program to Swap Two Numbers Without Third Variable
- C# Program to Convert Decimal to Binary
- C# Program to Convert a Number to Characters
- C# Program to Print Alphabet Triangle
- C# Program to Print Number Triangle
- C# Program to Generate Fibonacci Triangle
- C# New Features
- ADO.NET Tutorial
- ASP.NET Tutorial
- Partial Types in C#
- Iterators in C#
- Nullable Types in C#
- Delegate Covariance in C#
- Delegate Inference in C#
- Static Classes in C#
- Anonymous Types in C#
- Extension Methods in C#
- Query Expressions in C#
- Partial Methods in C#
- Implicitly Typed Local Variables
- Object and Collection Initializer
- Auto-Implemented Properties
- Dynamic Binding in C#
- Named and Optional Arguments
- Asynchronous Methods in C#
- Caller Info Attributes in C#
- Using Static Directive in C#
- Exception Filters in C#
- Await in Catch and Finally Blocks
- Auto Initialize Property in C#
- Default Values for Getter-Only Properties
- Expression-Bodied Members
- Null Propagator in C#
- String Interpolation
- nameof Operator in C#
- Local Functions in C#
- Digit Separator in C#
- Binary Literals in C#
- ref Keyword in C#
- Expression-Bodied Constructors and Finalizers
- Expression-Bodied Getters and Setters
- out Parameter in C#
- Async Main in C#
- Default Expression in C#