Express.js Tutorial | Learn Web Application Development with Node.js
Explore our Express.js Tutorial, covering basic to advanced concepts of Express.js, a minimal and flexible Node.js web application framework. Learn about routing, middleware, templating, RESTful APIs, authentication, error handling, debugging, and best practices. Get hands-on experience with Express installation, project setup, and real-world applications. Perfect for beginners and professionals aiming to build scalable web applications.
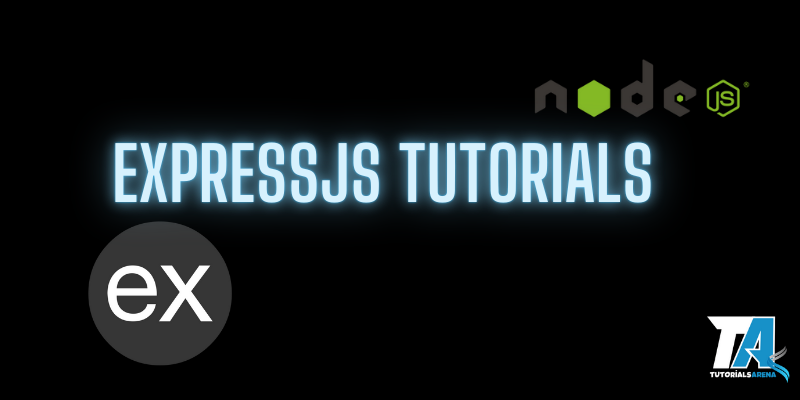
What is Express?
Express offers a minimalistic interface for building applications. It provides the essential tools to develop apps, and it is highly flexible due to the numerous modules available on npm (Node Package Manager) that can be easily integrated with Express.
Express was created by TJ Holowaychuk and is maintained by the Node.js Foundation along with many open-source contributors.
Why Choose Express?
Unlike other frameworks like Rails and Django, which have a prescribed way of building applications, Express offers more flexibility. It doesn't enforce a specific approach, giving developers the freedom to choose how to build their applications. This "unopinionated" nature makes Express highly adaptable and modular.
Pug (formerly Jade)
Pug is a concise and powerful templating language used with Express to generate HTML. Previously known as Jade, Pug provides the following features:
- Produces HTML efficiently
- Supports dynamic code for interactive pages
- Encourages reusability and the DRY (Don't Repeat Yourself) principle
Pug is one of the most popular templating languages used with Express for rendering dynamic HTML content.
MongoDB and Mongoose
MongoDB is an open-source, document-based database designed for scalability and ease of development. It stores data in flexible, JSON-like documents, which makes it a popular choice for web applications.
Mongoose is a Node.js library that provides a straightforward API for interacting with MongoDB databases. It simplifies data modeling, validation, and querying, making it easier to integrate MongoDB with an Express application.
With Express, MongoDB, and Mongoose, developers can build scalable and efficient web applications, from simple websites to complex backend services.
- ExpressJS Home
- Node.js Tutorial
- Installing Node.js
- Installing Node.js on Linux (Ubuntu, CentOS)
- First Node.js Program
- Node.js Console
- Node.js REPL (Read-Eval-Print Loop)
- npm (Node Package Manager)
- Node.js Command-Line Options
- Node.js Global Objects
- Node.js OS Module
- Node.js Timers
- Handling Errors in Node.js
- Node.js DNS Module
- Node.js Net Module
- Node.js Crypto Module
- Node.js TLS/SSL
- Node.js Debugger
- Node.js Process Module
- Node.js Child Processes
- Node.js Buffers
- Node.js Streams
- Node.js File System
- Node.js Path Module
- Node.js String Decoder
- Node.js Query String
- Node.js ZLIB
- Node.js Assertion Testing
- Node.js V8 Module
- Node.js Callbacks
- Node.js Events
- Node.js Punycode
- Node.js TTY
- Node.js Web Module
- NestJS
- Node.js MySQL Connection
- Node.js MySQL Create Database
- Node.js MySQL Create Table
- Node.js MySQL Insert Record
- Node.js MySQL Update Record
- Node.js MySQL Delete Record
- Node.js MySQL Select Record
- Node.js MySQL Select Unique Record
- Node.js MySQL Drop Table
- Node.js MongoDB Connection
- Node.js MongoDB Create Database
- Node.js MongoDB Create Collection
- Node.js MongoDB Insert
- Node.js MongoDB Select
- Node.js MongoDB Query
- Node.js MongoDB Sorting
- Node.js MongoDB Remove
- Node.js vs. AngularJS
- Node.js vs. Python
- Node.js vs. PHP
- Node.js vs. Java
- Node.js Process Environment Variables
- Node.js Semantic Versioning
- Node.js MCQ (Multiple Choice Questions)
- Express.js Tutorial
- Node.js Interview Questions
- AngularJS Interview Questions
- JavaScript Interview Questions
- jQuery Interview Questions
- Backbone.js Interview Questions
- Ember.js Interview Questions
- Neo4j Interview Questions