Java Continue Statement: Skipping Iterations in Loops
Learn about the continue
statement in Java, which is used in loop control structures to skip the current iteration and immediately jump to the next one. This statement enhances your ability to manage loop behavior efficiently, allowing you to fine-tune how your loops execute. Explore examples and best practices for using the continue
statement in your Java programs to improve code clarity and functionality.
Java - Continue Statement
Java Continue Statement
The continue
statement can be used in any loop control structure. It causes the loop to immediately jump to the next iteration.
In a for
loop, the continue
keyword causes control to jump to the update statement. In a while
loop or do/while
loop, control immediately jumps to the Boolean expression.
Syntax
The syntax of continue
is a single statement inside any loop:
Syntax
continue;
Java Continue Statement Flow Diagram
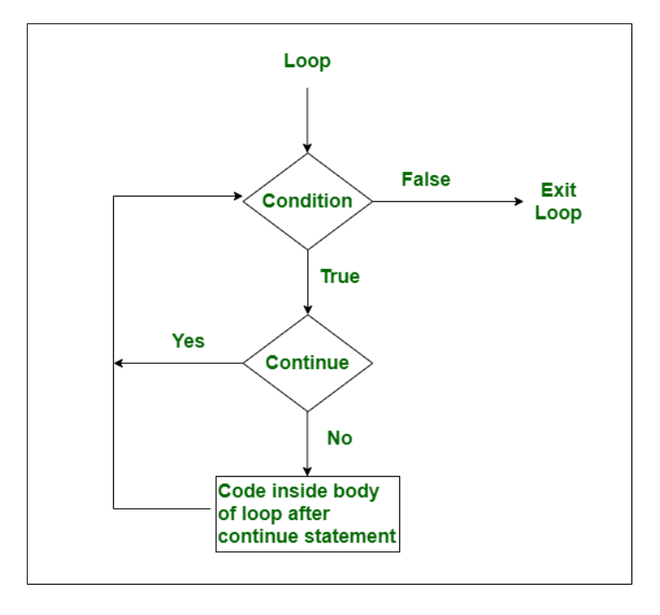
Examples
Example 1: Using continue with while loop
In this example, we demonstrate the use of a continue
statement to skip the element 15 in a while loop that prints elements from 10 to 19.
Example
public class Test {
public static void main(String args[]) {
int x = 10;
while( x < 20 ) {
x++;
if(x == 15){
continue;
}
System.out.print("value of x : " + x );
System.out.print("\n");
}
}
}
Output
value of x : 11
value of x : 12
value of x : 13
value of x : 14
value of x : 16
value of x : 17
value of x : 18
value of x : 19
value of x : 20
Example 2: Using continue with for loop
This example shows the use of a continue
statement within a for
loop to skip elements of an array during printing. An array of integers is created, and the loop iterates through it.
Example
public class Test {
public static void main(String args[]) {
int [] numbers = {10, 20, 30, 40, 50};
for(int index = 0; index < numbers.length; index++) {
if(numbers[index] == 30){
continue;
}
System.out.print("value of item : " + numbers[index] );
System.out.print("\n");
}
}
}
Output
value of item : 10
value of item : 20
value of item : 40
value of item : 50
Example 3: Using continue with do-while loop
This example demonstrates the use of a continue
statement to skip the element 15 in a do-while
loop that prints elements from 10 to 19.
Example
public class Test {
public static void main(String args[]) {
int x = 10;
do {
x++;
if(x == 15){
continue;
}
System.out.print("value of x : " + x );
System.out.print("\n");
} while( x < 20 );
}
}
Output
value of x : 11
value of x : 12
value of x : 13
value of x : 14
value of x : 16
value of x : 17
value of x : 18
value of x : 19
value of x : 20