Copying Dictionaries in Python: How to Create Independent Copies
Understand how to create a true copy of a dictionary in Python, as opposed to merely referencing the original. Learn to use the built-in copy()
method to make independent copies, ensuring changes in one dictionary do not affect the other.
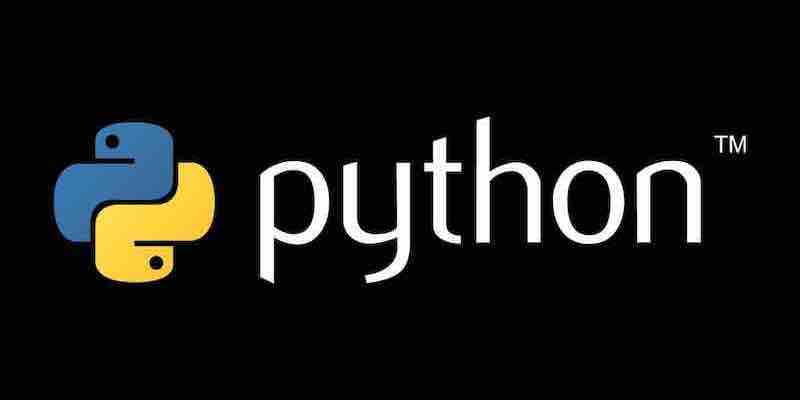
Copy a Dictionary
Dictionary cannot be copied by simply typing dict2 = dict1, because: dict2 will only be a reference to dict1, and changes made in dict1 will automatically be updated in dict2.
There are ways to make a copy, one way is to use the built-in Dictionary method copy()
.
Example
thisdict = {
"brand": "Ford",
"model": "Mustang",
"year": 1964
}
mydict = thisdict.copy()
print(mydict)
Output
{'brand': 'Ford', 'model': 'Mustang', 'year': 1964}
Another way to make a copy is to use the built-in function dict()
.
Example
# Make a copy of a dictionary with the dict() function
thisdict = {
"brand": "Ford",
"model": "Mustang",
"year": 1964
}
mydict = dict(thisdict)
print(mydict)
Output
{'brand': 'Ford', 'model': 'Mustang', 'year': 1964}